SOQCS Example 2: CNOT circuit.
We use SOQCS to simulate a CNOT circuit as described in [1].
[1] J L O’Brien, G J Pryde, A G White, T C Ralph, D Branning Demonstration of an all-optical quantum controlled-NOT gate. Nature 426:264 (2003)
[1]:
# Import libraries
import soqcs # Import SOQCS
from math import acos,sqrt,pi # Import mathematical functions
# Create a simulator
sim=soqcs.simulator()
Building a CNOT circuit
Here we build a circuit as described in [1]. Note that we are not defining the inputs like in example one. We left the channels open to indicate that we will append this circuit to the output of other circuit. Open channels helps to clarify the functionality of the circuit graphically like the barrier definition. A channel without any defined input will work in the same way.
[2]:
cnot = soqcs.qodev(2,6);
cnot.open_channel(0)
cnot.open_channel(1)
cnot.open_channel(2)
cnot.open_channel(3)
cnot.open_channel(4)
cnot.open_channel(5)
cnot.separator()
cnot.beamsplitter(3,4, -45.0,0.0)
cnot.separator()
cnot.beamsplitter(0,1,180*acos(1.0/sqrt(3.0))/pi,0.0)
cnot.beamsplitter(2,3,180*acos(1.0/sqrt(3.0))/pi,0.0)
cnot.beamsplitter(4,5,180*acos(1.0/sqrt(3.0))/pi,0.0)
cnot.separator()
cnot.beamsplitter(3,4, -45.0,0.0)
cnot.separator()
cnot.phase_shifter(1, 180)
cnot.phase_shifter(3, 180)
cnot.separator()
cnot .detector(0,0)
cnot.detector(1)
cnot.detector(2)
cnot.detector(3)
cnot.detector(4)
cnot.detector(5,0)
cnot.show(sizexy=80,depth=13)
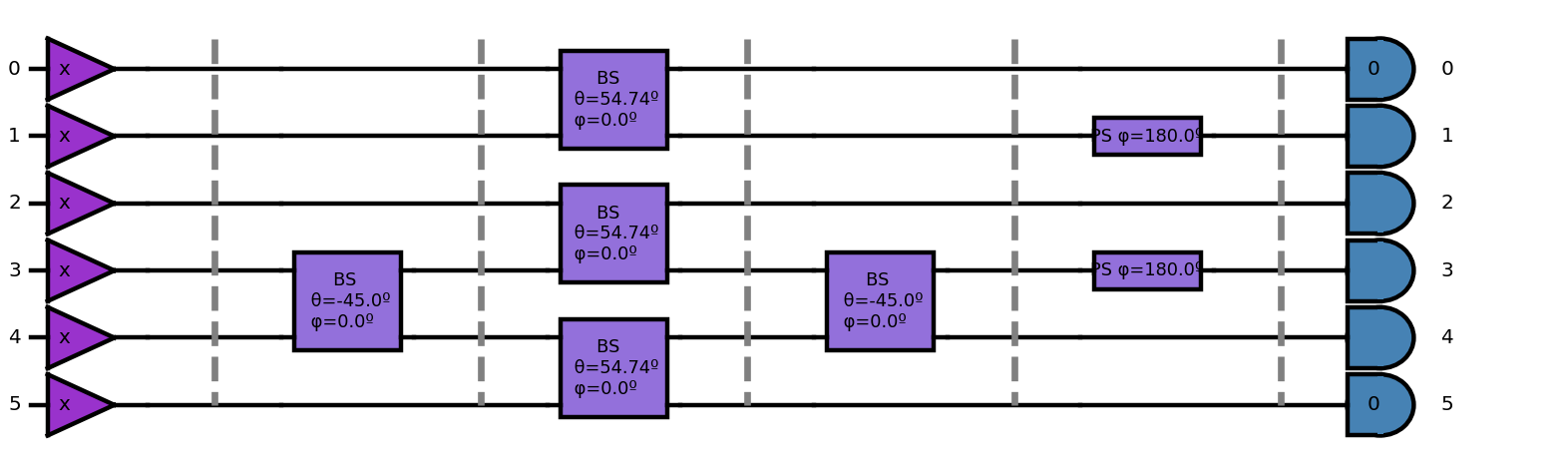
Qubit declaration
We will relate the input channels with the qubit definition. In this case, we use a path encoded photon initialization. A pair of channels define a qubit. If the occupation of that pair of channels is “01” then the qubit value is “0”. If the occupation is “10” then the value is “1”. Below we define a 2xn matrix with as many columns as qubits. The first row determines the leftmost value while evaluating occupations.
[3]:
qmap=[[1, 3],
[2, 4]]
Example with | 1, 0 > input.
Below we create a circuit and we define the input adding the photons to the circuit. The initialization can be done directly from the qubit values. In this case the list qlist=[1,0]. Channels that do not belong to a qubit definition are the ancilla channels. We initialize both channel 0 and 5 to zero with the list ancilla=[0,0] The values of this list are assigned to the channels in order by their channel number. Once the circuit is initialized we append the previous circuit with the gate definitions.
[4]:
cnot10=soqcs.qodev(2,6); # Create a circuit
cnot10.qubits(qlist=[1,0],ancilla=[0,0],qmap=qmap) # Define the input
cnot10 << cnot # Append the gates
cnot10.show(depth=20,slin=1) # Show the resulting circuit.

Simulation and plot of the output. The output is a photonic one therefore we need to traslate this output into qubit encoding before the plot.
[5]:
outcome=sim.run(cnot10) # Run the simulation
encoded=outcome.translate(qmap, cnot10) # Encode the output
encoded.show(sizex=5,dpi=70) # Plot the result
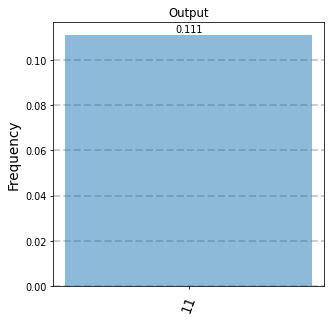
Additional results
Here we repeat the same process for the rest of the inputs.
|0, 0 > input
[6]:
cnot00=soqcs.qodev(2,6);
cnot00.qubits([0,0],[0,0],qmap)
cnot00 << cnot
outcome=sim.run(cnot00)
encoded=outcome.translate(qmap, cnot10)
encoded.show(sizex=5,dpi=70)
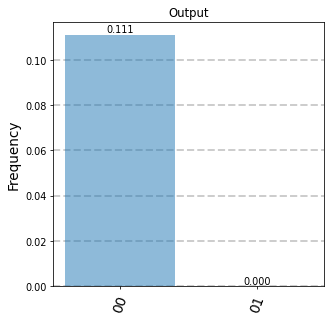
|0, 1 > input
[7]:
cnot01=soqcs.qodev(2,6);
cnot01.qubits([0,1],[0,0],qmap)
cnot01 << cnot
outcome=sim.run(cnot01)
encoded=outcome.translate(qmap, cnot10)
encoded.show(sizex=5,dpi=70)
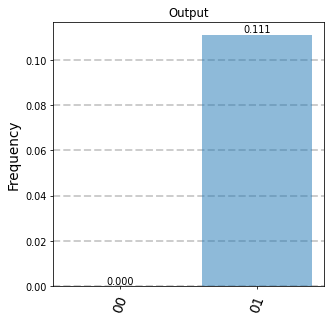
|1, 1 > input
[8]:
cnot11=soqcs.qodev(2,6);
cnot11.qubits([1,1],[0,0],qmap)
cnot11 << cnot
outcome=sim.run(cnot11)
encoded=outcome.translate(qmap, cnot10)
encoded.show(sizex=5,dpi=70)
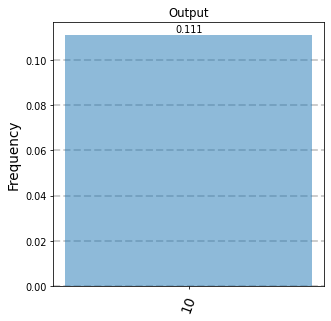
THIS CODE IS PART OF SOQCS
Copyright: Copyright © 2023 National University of Ireland Maynooth, Maynooth University. All rights reserved. The contents and use of this document and the related code are subject to the licence terms detailed in LICENCE.txt